Python: best_practices[2]
- Alex
- Feb 21, 2021
- 2 min read
Updated: Sep 24, 2021
Write better Python code in the future.
Part 3 of our snippets and learning moments in Python.
Topics I recently remembered or picked up. Here is the recollection of all this posts:
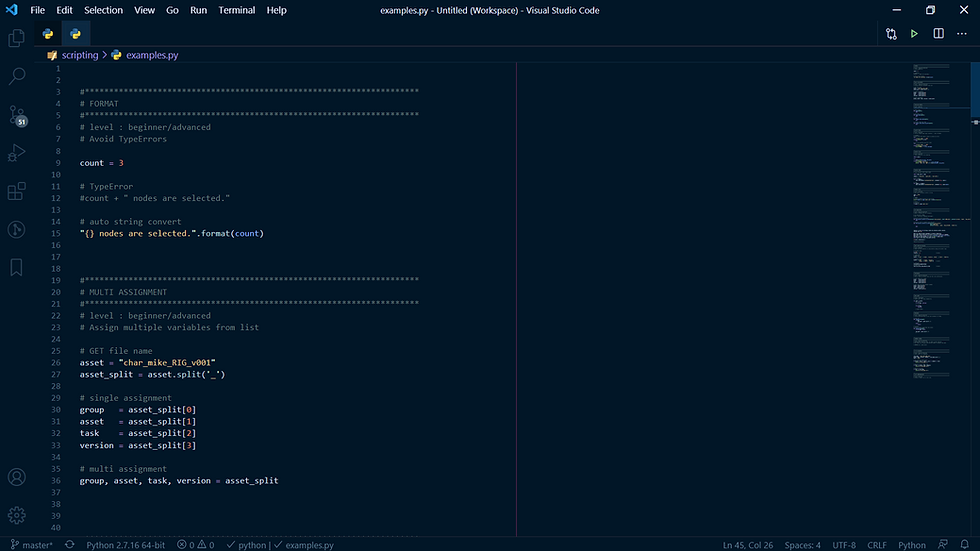
DEBUGGING
The process of solving a bug/error which takes up to 75% of your time.
Master debugging to master scripting.
"Frame: " + 1001
# Traceback (most recent call last):
# File "/server/week_05.py", line 32, in <module>
# "Frame: " + 1001
# TypeError: cannot concatenate 'str' and 'int' objects
# EXPLAIN
# [EXCEPTION] Code stops and the traceback prints out the interpreter issue
# [WHERE] file path, line 32, module
# [WHAT] TypeError: cannot concatenate 'str' and 'int' objects
# Cannot combine (+) a string and an integer
SAME LINE STATEMENTS
Semicolons can be used to delimit statements (see C++, MEL, ...)
when you must put multiple statements in the same line.
# idiomatic
print("test")
print("works too")
# delimit statement
print("test"); print("works too")
# WARNING: Bad style!!!
# USE CASE: UI button commands
# A call needs to be converted into a multi statement string
# cmds.button(label="Start Tool", command="import tool; tool.start()")
ENUMERATE
Get the index and value of a sequence.
assets = ['mike', 'sulley', 'camera']
enum_assets = enumerate(assets)
# [(0, 'mike'), (1, 'sulley'), (2, 'camera')]
for index, value in enum_assets:
assets[index] = value + '_001'
MULTI RETURN
Return multiple values (e.g. enumerate).
def get_type_task(file_name):
file_split = file_name.split('_')
return file_split[0], file_split[1]
file_name = 'char_mike_RIG_v001.ma'
group, asset = get_type_task(file_name)
SLICE
Limit a sequence with seq[first:last].
# slice list
assets = ["mike", "sulley", "announcer", "table"]
assets[1:3] # ['sulley', 'announcer']
# slice string
character = "mike_001"
character[-3:] # 001
WEBBROWSER
Opens anything in your default browser or OS.
import webbrowser
# WEBSITE
webbrowser.open("https://www.alexanderrichtertd.com")
# IMG & VIDEOS
webbrowser.open("/server/image.png")
webbrowser.open("/server/video.mp4")
# SCRIPTS | LOGS | ...
webbrowser.open("/server/script.py")
# DIRECTORIES
webbrowser.open("/server/project")
"" vs ''
There is no difference between double and single quotes but we careful mixing them.
# ERROR: without escape characters
print("Are you really a "Superhero"?")
# Typical: Integrate "" in ''
print('Are you really a "Superhero"?')
# Are you really a "Superhero"?
# Or integrate '' in ""
print("Are you really a 'Superhero'?")
# Are you really a 'Superhero'?
ESCAPE CHARACTERS
Backslash (\) allows escape characters with special meaning
e.g. newline (\n), backslash (\\), quote (\' or \")
# ERROR: without escape characters
print("Are you really a "Superhero"?")
# Alternative: Mixing '' and ""
print('Are you really a "Superhero"?')
# Quotations
print('Are you really a \'Superhero\'?')
print("Are you really a \"Superhero\"?")
# Add newline between two sentences
# n is an escape character and will not be displayed as \n
print("Hello?\nCan you read this?")
# Hello?
# Can you read this?
- Alex
PS. For more content subscribe to my Newsletter.
Comments